在現代Web開發中,發送電子郵件是許多應用程序的基本功能之一。PHP作為一種廣泛使用的服務器端腳本語言,提供了簡單而有效的方法來實現電子郵件發送功能。為了更復雜的需求,例如發送帶有附件的電子郵件,PHP提供了多種解決方案來實現這一點。在方維網站建設中,我們將深入探討如何使用PHP程序發送帶附件的郵件,涵蓋從最基礎的mail()函數到使用功能更為強大的PHPMailer庫的方法。
### 使用PHP的mail()函數發送郵件
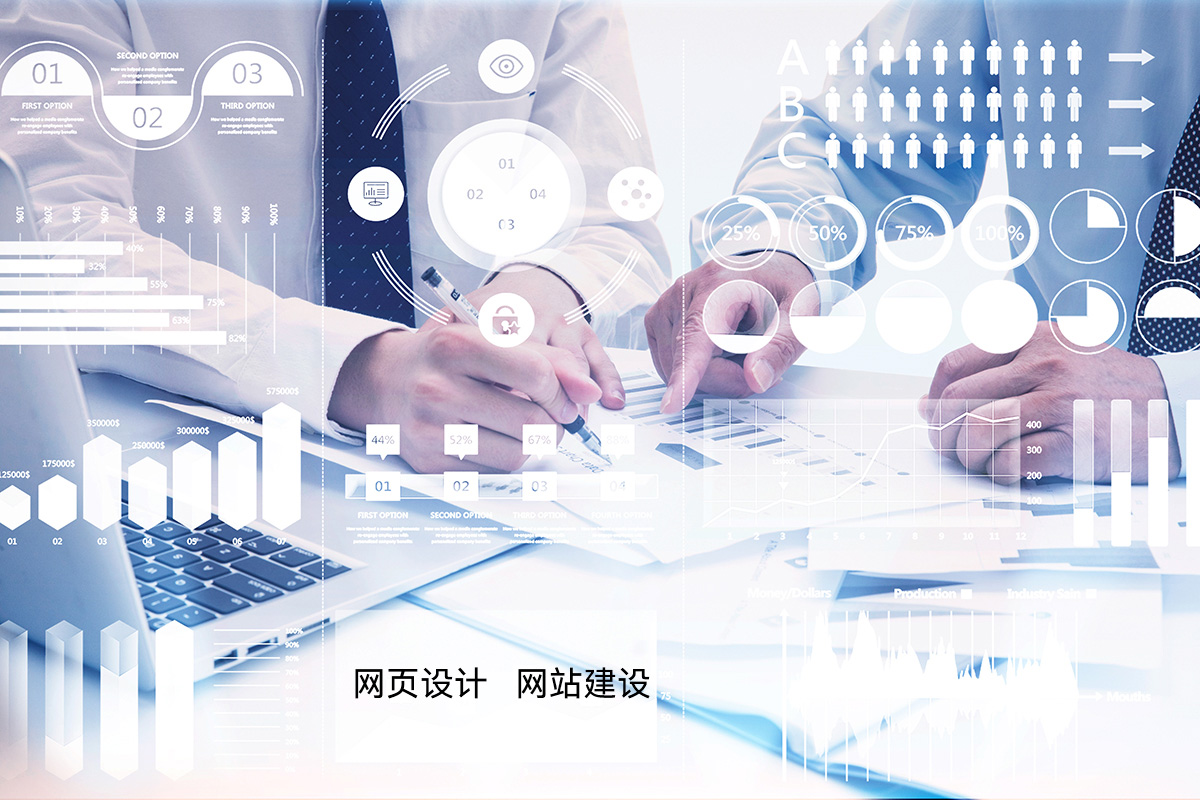
PHP內置的`mail()`函數提供了基本的發送電子郵件的功能。其語法如下:
```php
bool mail(string $to, string $subject, string $message, string $headers, string $parameters);
```
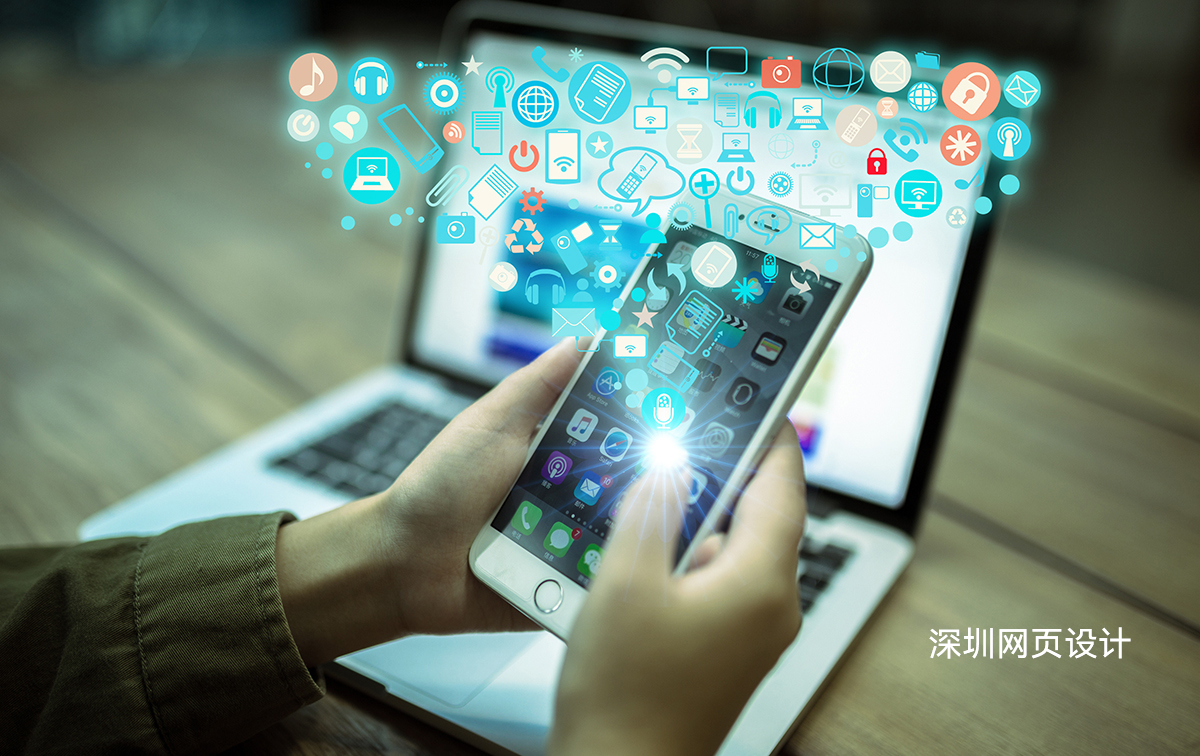
雖然`mail()`函數簡單易用,但它在處理附件方面顯得有些局限。為了發送郵件帶附件,我們需要手動構建電子郵件的MIME格式。
### 構造MIME郵件以發送附件
要發送帶附件的電子郵件,我們需要手動設置郵件的頭部信息以支持MIME(Multipurpose Internet Mail Extensions)格式。這允許我們發送非文方維網站建設件作為附件。以下是一個示例代碼展示了如何使用PHP的`mail()`函數發送帶附件的郵件:
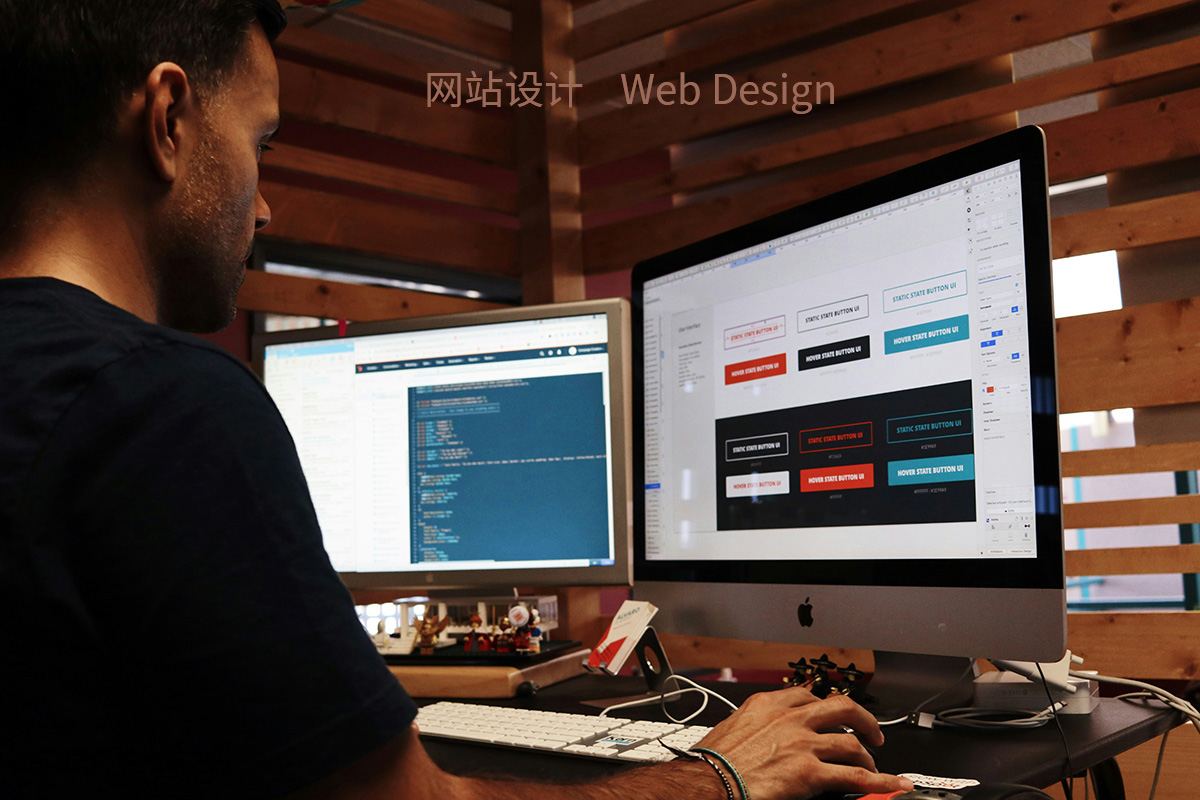
```php
$to = 'recipient@example.com';
$subject = 'Here is your attachment';
$message = 'Please see the attached file.';
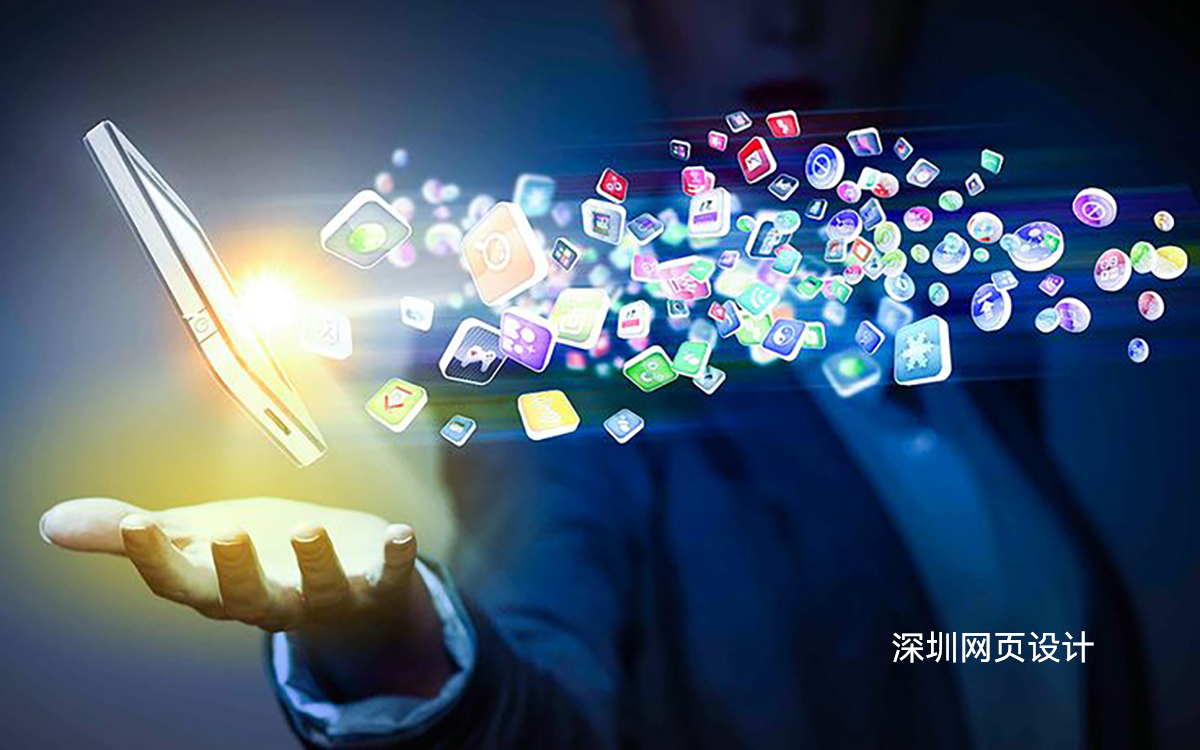
$file = '/path/to/your/file.txt';
$fileData = file_get_contents($file);
$fileType = mime_content_type($file);
$fileName = basename($file);
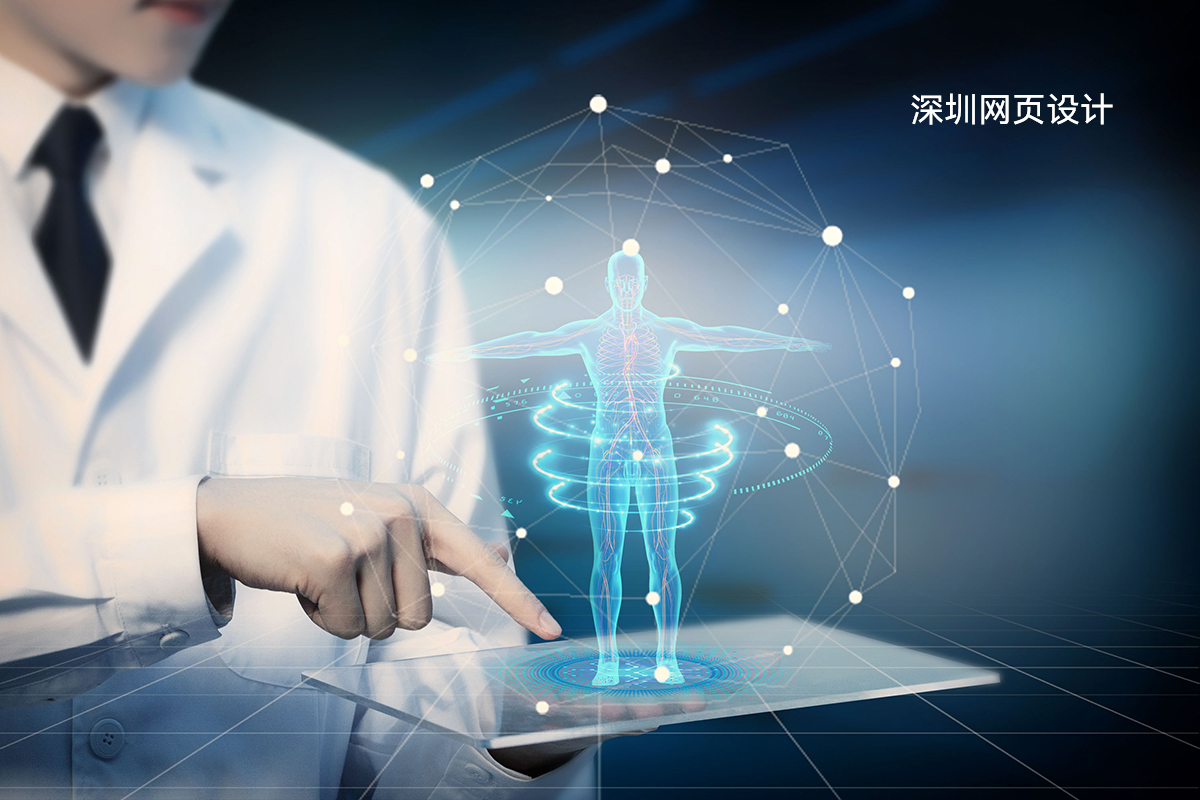
$boundary = md5(time());
$headers = "MIME-Version: 1.0\r\n";
$headers .= "From: sender@example.com\r\n";
$headers .= "Reply-To: sender@example.com\r\n";
$headers .= "Content-Type: multipart/mixed; boundary=\"$boundary\"";

$body = "--$boundary\r\n";
$body .= "Content-Type: text/plain; charset=UTF-8\r\n";
$body .= "Content-Transfer-Encoding: 7bit\r\n";
$body .= "\r\n";
$body .= $message . "\r\n";
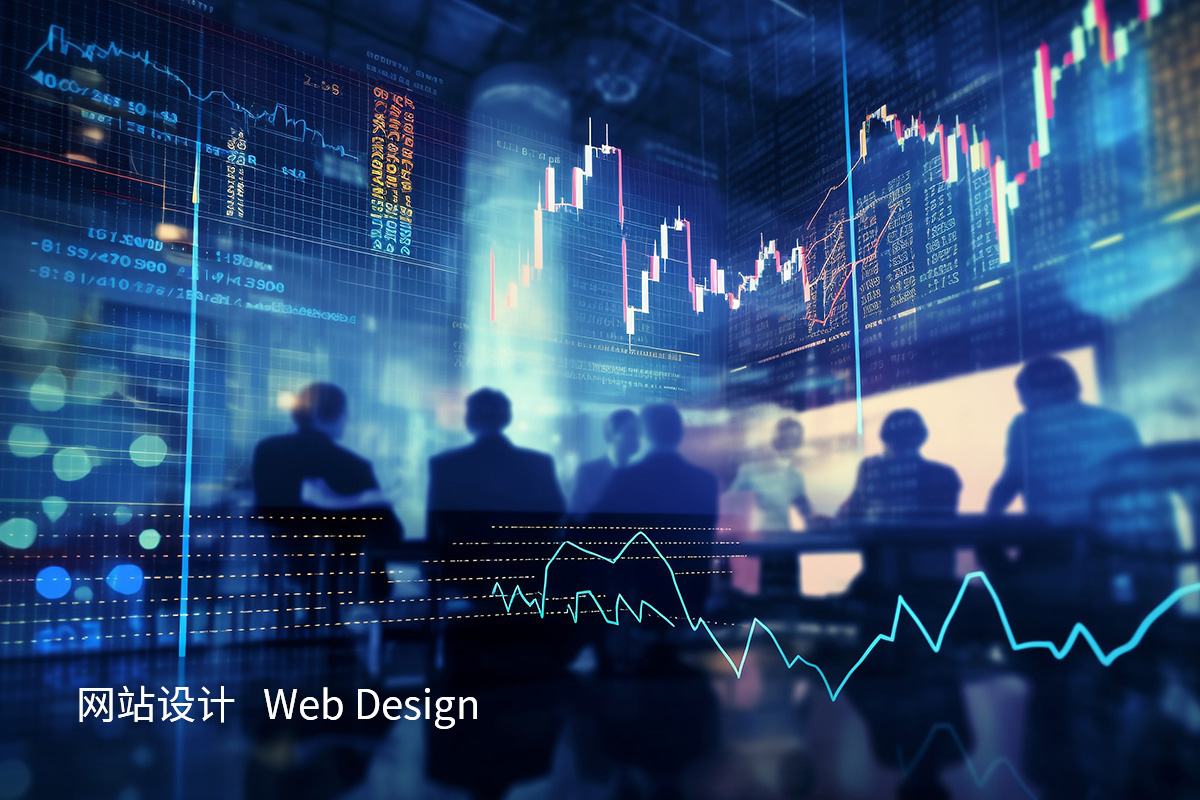
$body .= "--$boundary\r\n";
$body .= "Content-Type: $fileType; name=\"$fileName\"\r\n";
$body .= "Content-Disposition: attachment; filename=\"$fileName\"\r\n";
$body .= "Content-Transfer-Encoding: base64\r\n";
$body .= "\r\n";
$body .= chunk_split(base64_encode($fileData)) . "\r\n";
$body .= "--$boundary--";
mail($to, $subject, $body, $headers);
?>
```
在這份示例代碼中,我們手動構建了郵件消息和附件的MIME邊界,并對附件進行了Base64編碼以適合郵件傳輸。這種方法雖然可以實現功能,但代碼復雜且不易維護。因此,我們推薦使用更現代化的庫,如PHPMailer以簡化流程。
### 使用PHPMailer發送帶附件的郵件
PHPMailer是一個廣受歡迎的PHP郵件發送庫,它簡化了郵件發送過程并支持各種附加功能,如SMTP、HTML郵件格式、附件等。下面是如何用PHPMailer發送帶附件郵件的步驟:
1. **安裝PHPMailer**:我們可以使用Composer來安裝PHPMailer,這是管理PHP依賴項的首選方法。運行以下命令來安裝:
```bash
composer require phpmailer/phpmailer
```
2. **發送郵件代碼示例**:
```php
use PHPMailer\PHPMailer\PHPMailer;
use PHPMailer\PHPMailer\Exception;
require 'vendor/autoload.php';
$mail = new PHPMailer(true);
try {
//服務器設置
$mail->SMTPDebug = 2;
$mail->isSMTP();
$mail->Host = 'smtp.example.com';
$mail->SMTPAuth = true;
$mail->Username = 'your-email@example.com';
$mail->Password = 'your-email-password';
$mail->SMTPSecure = PHPMailer::ENCRYPTION_STARTTLS;
$mail->Port = 587;
//收件人設置
$mail->setFrom('from@example.com', 'Mailer');
$mail->addAddress('recipient@example.com', 'Joe User');
//附件
$mail->addAttachment('/path/to/file.txt');
//內容
$mail->isHTML(true);
$mail->Subject = 'Here is the subject';
$mail->Body = 'This is the HTML message body
in bold!';
$mail->AltBody = 'This is the body in plain text for non-HTML mail clients';
$mail->send();
echo 'Message has been sent';
} catch (Exception $e) {
echo "Message could not be sent. Mailer Error: {$mail->ErrorInfo}";
}
?>
```
在這個例子中,PHPMailer's強大功能顯而易見。我們只需進行簡短的配置,就能實現復雜的郵件發送需求。PHPMailer通過內置的SMTP支持和自動處理MIME,使得發送郵件帶附件變得更加高效、快捷。
### 為什么選擇PHPMailer
1. **易用性**:PHPMailer's接口設計簡潔流暢,無需手動編寫復雜的郵件頭信息。
2. **功能豐富**:支持SMTP、安全連接、HTML格式、附件、多語言等眾多功能。
3. **社區支持**:PHPMailer有一個活躍的開發社區,提供快速的更新和支持。
4. **強大的錯誤處理**:PHPMailer通過異常處理機制提供詳細的錯誤信息,實現更好的錯誤管理。
### 總結
發送帶附件的電子郵件是許多應用程序的基本需求。雖然PHP的`mail()`函數足以滿足簡單的需求,但使用PHPMailer這樣的庫可以大大簡化代碼,提高可讀性和維護性。通過PHPMailer的使用,開發者可以快速實現復雜的郵件功能,無需過多關注底層實現細節。
不論選擇哪種方法,理解郵件發送的基礎原理和不同方法的優缺點都將幫助開發者根據項目的具體需求做出最佳的選擇。希望方維網站建設能夠為使用PHP程序發送郵件提供一個清晰的指南。